Powershell Basics - You Can't Dodge a Blue Shell
The basics of Powershell are important to understand if you want to write a script that won't have you pulling your hair out halfway through. Understanding the basics will set you up for success going forward.
What is Powershell?
Powershell is both a command-line shell and a scripting language, specifically targeted towards system administrators. This means that you can write scripts to run, or actively use the language in a command line environment. Powershell is available by default on all Windows OSes, and can be installed on MacOS and Linux as well.
Powershell operates by processing objects, which are comprised of properties (or characteristics) and methods (actions that be performed on the object).
Where do I write Powershell?
In Windows you can use Powershell interactively in a Powershell Prompt, which is accessed by opening Windows Powershell application. This is best used for running built in or added commands, or running scripts you've previously written.
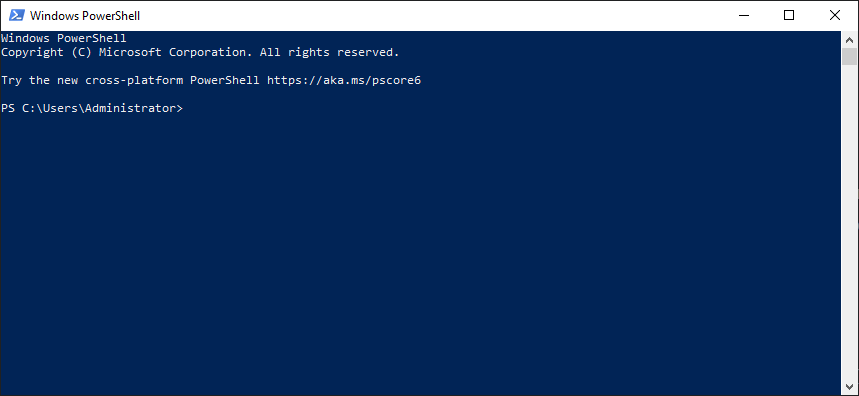
The Powershell ISE (Integrated Scripting Environment) is built into Windows and features a script editing screen, as well as the now-familiar blue terminal in a split screen format. What you write will remain in the top script editing screen, and you can optionally run either the entire tab as a single script, or limit which code is executed to only selected lines. Any output will appear in the blue console. You can also run any Powershell commands by typing them into the console directly, although they will not be as easily accessible to run again if needed.
In the script editing screen, text will be color-coded based on the contents. To even further increase readability, selecting a bracket or parenthesis will locate and highlight the matching bracket or parenthesis if there is one.
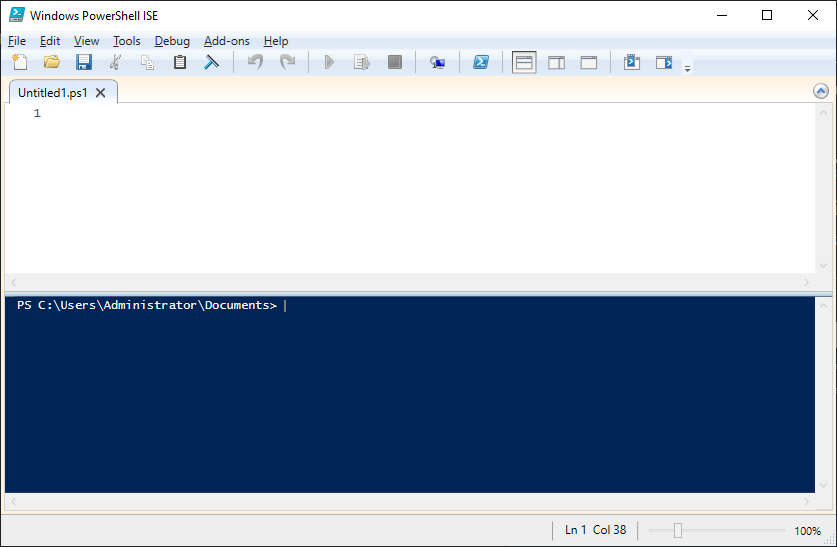
Another option is VS Code with the Powershell Extension. VS Code is an IDE (Integrated Development Environment), and is used for writing code in more languages than just Powershell. It has several additional features that make it very useful for writing scripts over the Powershell ISE.
In addition to the split screen between the IDE and the Console (both in black below), you have the option to open a file explorer view which makes it very easy to work with multiple files concurrently.
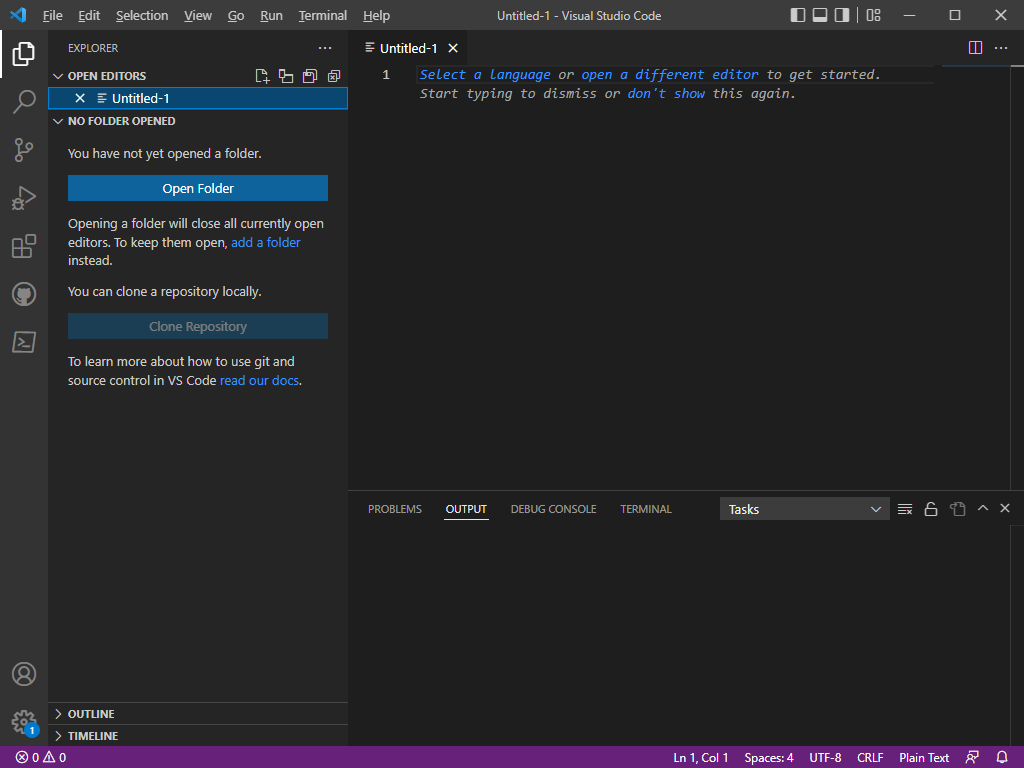
If you are working on multiple files you can open them up in a split screen. This works great if you're referencing something you did in another file, or are making changes in multiple files at once.
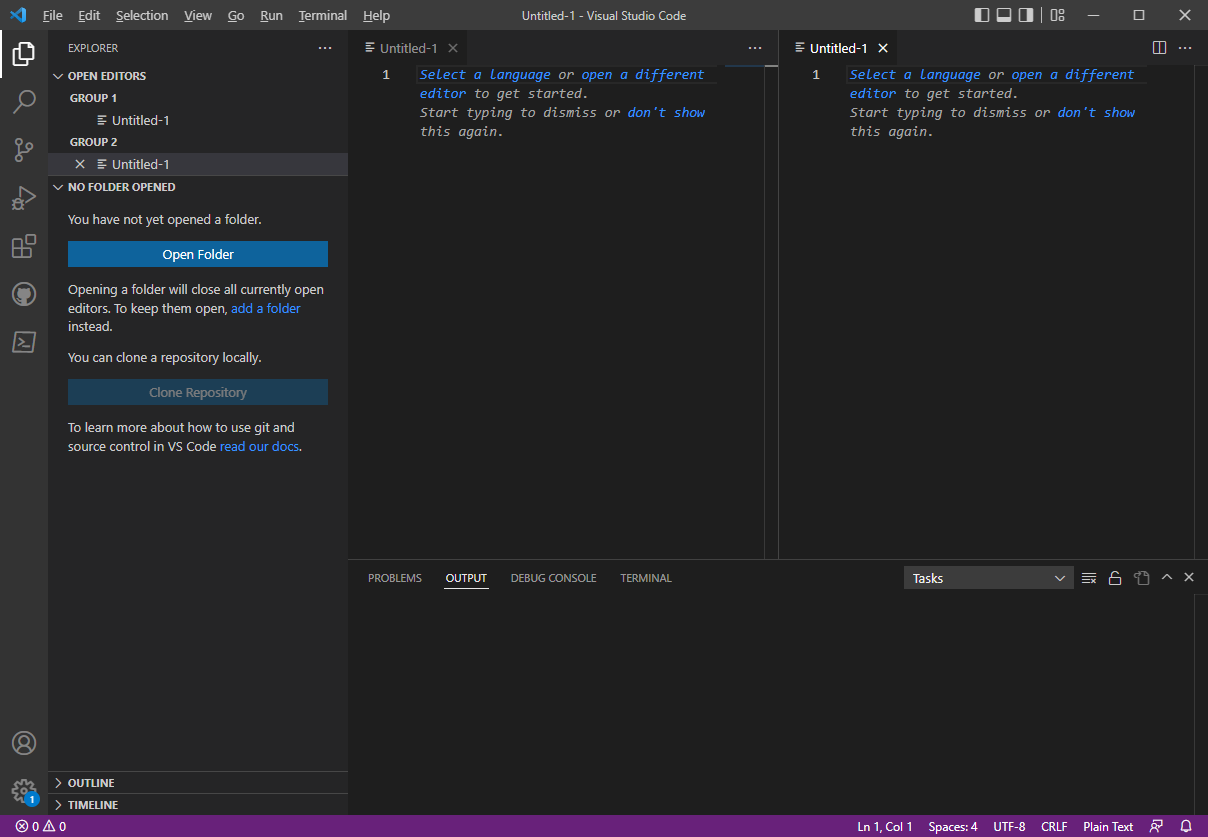
Not only will VS Code color code your code, it color codes your brackets and parentheses so it is easy to identify their pairs.
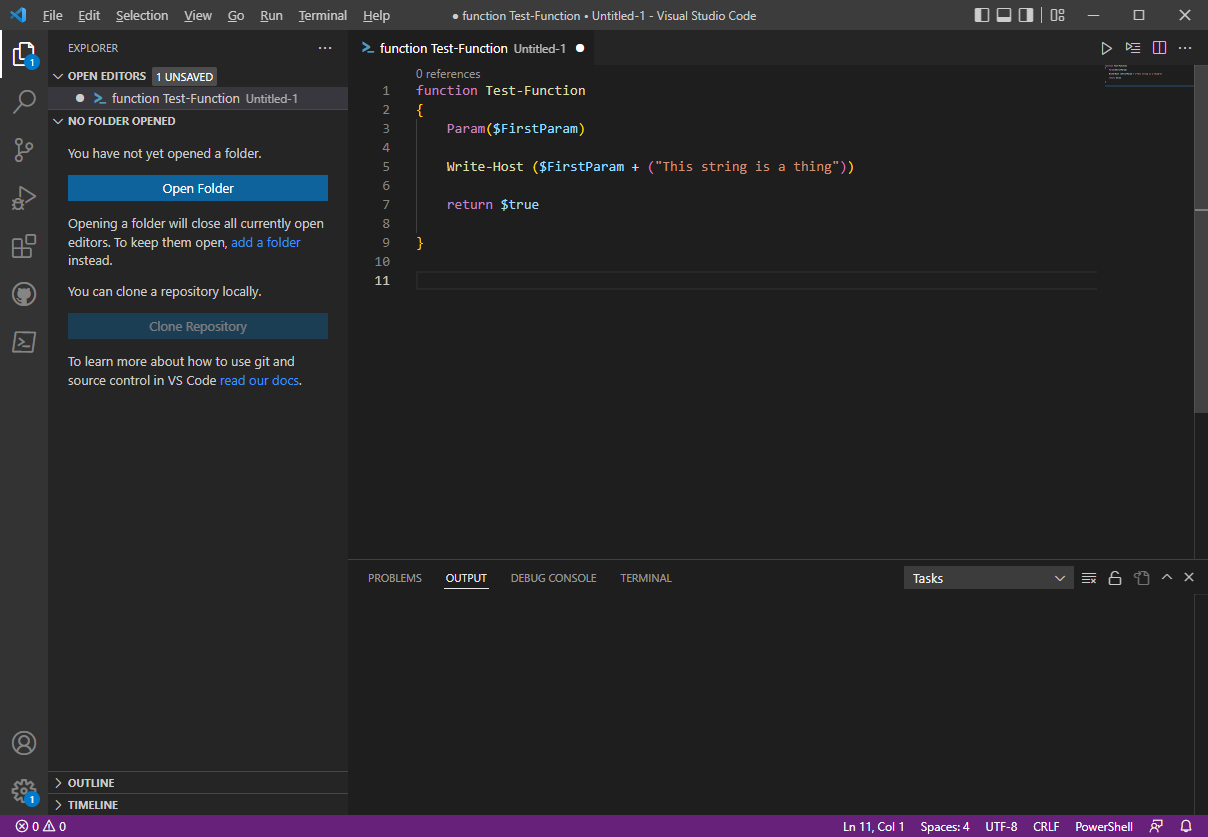
In both VS Code and Powershell ISE, the palette of the color coding is editable so that you can choose what color something is if you don't like the default. You can see the very colorful options that come with the default Dark Mode in the above image.
You have the option to run your whole script, or just a selected part of it in either VS Code or the Powershell ISE. Pressing F5 on your keyboard, or pressing the Play Arrow (Upper left in VS Code, Upper Middle in Powershell ISE) will run your whole script. Pressing F8 or pressing the smaller play arrow with the lines/page behind it will only run what you've selected, or if you have nothing selected the line that your cursor is on.
What goes into a Powershell Script?
A Powershell script consists of variables, loops, conditions, flow-control, and functions. A brief description of each is below, but each will be covered more deeply in a dedicated article.
Comments
Comments in your code are important so that you can describe what a particular part of your code is doing. That way when someone else looks at your code, they don't have to try and guess what you were thinking. More importantly, when you look at your code six months down the line, you don't have to try and guess what you were thinking.
#This is a single line comment in Powershell
<#
This is a
Multi-Line Comment
In Powershell
#>
Variables
A variable is a storage location for some value (a single value or an object). Variables are unique to the running session of Powershell and not accessible outside the code that is currently running with some exceptions.
#Declare a Variable and write it to the console
$Variable = "This is a variable."
Write-Host $Variable
Loops
Loops are used to iterate over a group of data and perform the same actions to each individual item within that group until a certain condition is met.
#Loop 10 times and output the number the loop is on
for($i = 1; $i -le 10; $i++)
{
Write-Host "This is loop numnber" $i
}
Conditions
Conditional statements, or decision making statements, are used to make a choice on what piece of code to run based on an evaluation. The simplest version of this is the If/Else statement: If Condition is met, do A - Else, do B.
#Declare a variable to be equal to $true, if it is True, Output "Kangaroo", if it isn't, Output "Koala"
#Try chaning the Variable to be equal to $false
$Variable = $true
if($Variable -eq $true)
{
Write-Host "Kangaroo"
}
else
{
Write-Host "Koala"
}
Flow Control
Flow control is what allows you to define a function one time and then call that function as needed throughout the rest of the script. Rather than having to code out everything that needs to happen in the order that it happens, you can separate pieces of code to simplify your code and make it easier to write and read.
Functions
Functions are pieces of code that perform a designated set of predefined actions. These can be built into Powershell, added via a module, or written by you.
#Define a function and then call it multiple times
function Roll-Dice
{
return Get-Random -Maximum 7 -Minimum 1
}
Roll-Dice
Roll-Dice
Roll-Dice
One Last Thing - Scope
In simple terms, Scope refers to where a variable can be seen and interacted with. If you define a function and declare a variable in that function, the variable only exists within that function and ceases to exist when the function finishes. Similarly, when declaring a variable within a script, any functions you define within that script can interact with that variable (this is generally a bad practice), but anything outside of that script (such as another script) cannot. Scope is important to understand so you don't accidentally overwrite your variables with unwanted values, and so that you can properly access your variables and their values where and when you need to. For example, two separate functions in your code can each declare their own variable named $Input, but because of Scope the $Input variable from Function A is completely isolated and independent from the $Input variable in Function B and they won't interfere with each other.
#Define two functions, both using $Result as a variable name
function Roll-Dice
{
#This $Result only exists when Roll-Dice is called and being processed
$Result = Get-Random -Maximum 7 -Minimum 1
return $Result
}
function Draw-Card
{
#This $Result only exists when Draw-Card is called and being processed
$Result = Get-Random -Maximum 14 -Minimum 2
return $Result
}